Designing a Scalable URL Shortener Service on AWS: A Comprehensive Guide
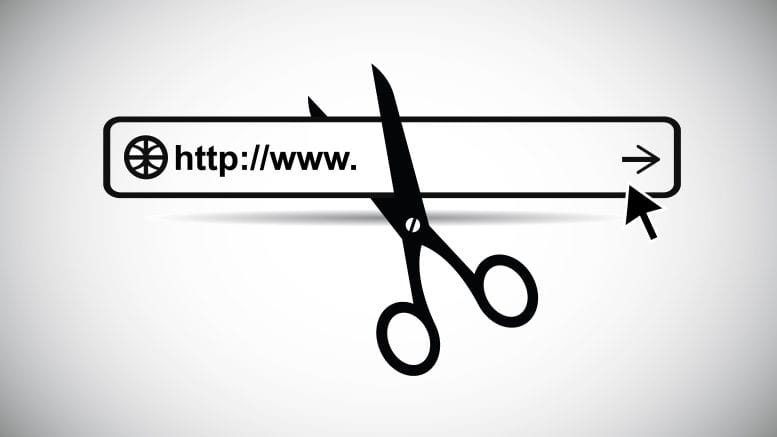
URL shortening services have become an essential part of our digital ecosystem, making long, unwieldy URLs easier to share across platforms with character limitations and improving the overall user experience. In this comprehensive guide, we'll explore how to design and implement a highly scalable, robust URL shortener service using AWS services. Whether you're looking to build your own private URL shortener for business purposes or simply want to understand the architecture behind such systems, this post will provide you with detailed insights and implementation guidance.
Understanding URL Shortener Systems
Before diving into the technical aspects, let's understand what URL shorteners do and why they are useful. URL shorteners take a long URL as input and generate a significantly shorter URL that redirects to the original destination. These services are valuable in several scenarios:
- When sharing links on platforms with character limitations (like Twitter/X)
- For tracking click metrics and user engagement
- For improving the aesthetics of shared links in marketing materials
- For branding purposes, where companies want a consistent URL pattern
System Requirements
As with any system design, we begin by defining clear requirements. Let's break these down into functional and non-functional requirements.
Functional Requirements
The core functionality of our URL shortener service includes:
- URL Shortening: The system must take a long URL as input and return a shortened URL.
- URL Redirection: When users access the shortened URL, they should be redirected to the original long URL.
Additional features that enhance the system's value include:
- Custom URL Creation: Allowing users to define their own custom short URLs for branding purposes.
- Analytics: Tracking metrics like the most popular URLs, geographic distribution of clicks, and referral sources.
- Expiration Settings: Enabling URLs to automatically expire after a predefined period.
- API Access: Providing programmatic access for third-party integrations.
Non-Functional Requirements
Equally important are the quality attributes that determine how well the system operates:
- High Availability: The system should maintain high uptime, as users expect links to work consistently.
- Low Latency: Both URL creation and redirection should happen quickly to ensure a smooth user experience.
- Scalability: The system should handle growing numbers of requests without performance degradation.
- Security: Protection against malicious use and ensuring data privacy.
- Data Durability: URLs and their mappings must be preserved reliably for their intended lifespan.
System Scale Considerations
Understanding the scale at which your URL shortener will operate helps in making appropriate design decisions. Here are some key metrics to consider:
- URL Creation Rate: How many new short URLs will be generated per second?
- URL Redirection Rate: How many redirect requests will the system handle per second?
- Storage Requirements: How much data storage will be needed to maintain all URL mappings?
For our design, let's assume the following reasonable estimates:
- 1,000 new URL creation requests per second
- 20,000 URL redirection requests per second
- URLs remain in the system for an average of one year
Based on these assumptions, we can calculate that our system will need to store approximately 31.53 billion URLs in a year (1,000 × 60 × 60 × 24 × 365).
URL Shortening Algorithms
The core functionality of our service depends on efficiently generating short, unique identifiers for long URLs. Let's explore the main approaches:
Hashing Approach
One method involves using cryptographic hash functions like MD5 or SHA-256 to generate a fixed-length hash of the original URL. While simple to implement, this approach has a significant drawback: potential hash collisions where different long URLs produce the same shortened URL.
Unique ID Generation
A more robust approach uses an incremental counter or a unique ID generation service. This method:
- Eliminates collision concerns
- Can be optimized for performance
- Avoids redundant database lookups
For optimal performance and scalability, we can implement a dedicated Key Generation Service (KGS) that pre-generates unique IDs and provides them to the URL shortener service when needed. This separation of concerns allows each component to focus on its specific role:
- The KGS handles the creation and management of unique identifiers
- The URL shortener service maps these identifiers to long URLs and handles redirection
Determining the Optimal Short URL Length
To ensure our system can handle the required scale, we need to determine the appropriate length for our short URLs. The character set for our short URLs includes:
- Numbers (0-9): 10 characters
- Lowercase letters (a-z): 26 characters
- Uppercase letters (A-Z): 26 characters
This gives us 62 unique characters to use. The number of possible unique URLs for different lengths is:
- Length 6: 62^6 = 56.8 billion
- Length 7: 62^7 = 3.5 trillion
- Length 8: 62^8 = 218.3 trillion
Based on our scale requirement of 31.53 billion URLs per year, a URL length of 7 characters provides ample capacity while keeping URLs reasonably short.
Storage Requirements
Each URL mapping requires storage for:
- Short URL (7 characters): 7 bytes
- Long URL (average 100 characters): 100 bytes
- Expiration date: 8 bytes
- Metadata (user information, preferences): Approximately 1 KB
At a conservative estimate of 1 KB per URL mapping, storing 31.53 billion URLs would require approximately 29.37 TB of storage per year.
AWS Architecture Design
Now, let's design our URL shortener service using AWS services. We'll present two approaches: a Day 0 architecture for getting started quickly and a more robust Day N architecture for scaling to millions of users.
Day 0 Architecture: Getting Started
For rapid deployment and validation, we can implement a serverless architecture using these AWS services:
- Amazon API Gateway: Handles all HTTP requests, both for creating short URLs and redirecting users
- AWS Lambda: Contains the business logic for URL shortening and redirection
- Amazon DynamoDB: Stores the mappings between short and long URLs
- Amazon ElastiCache (Redis): Caches frequently accessed URL mappings for faster response
- AWS CloudWatch: Monitors system performance and logs
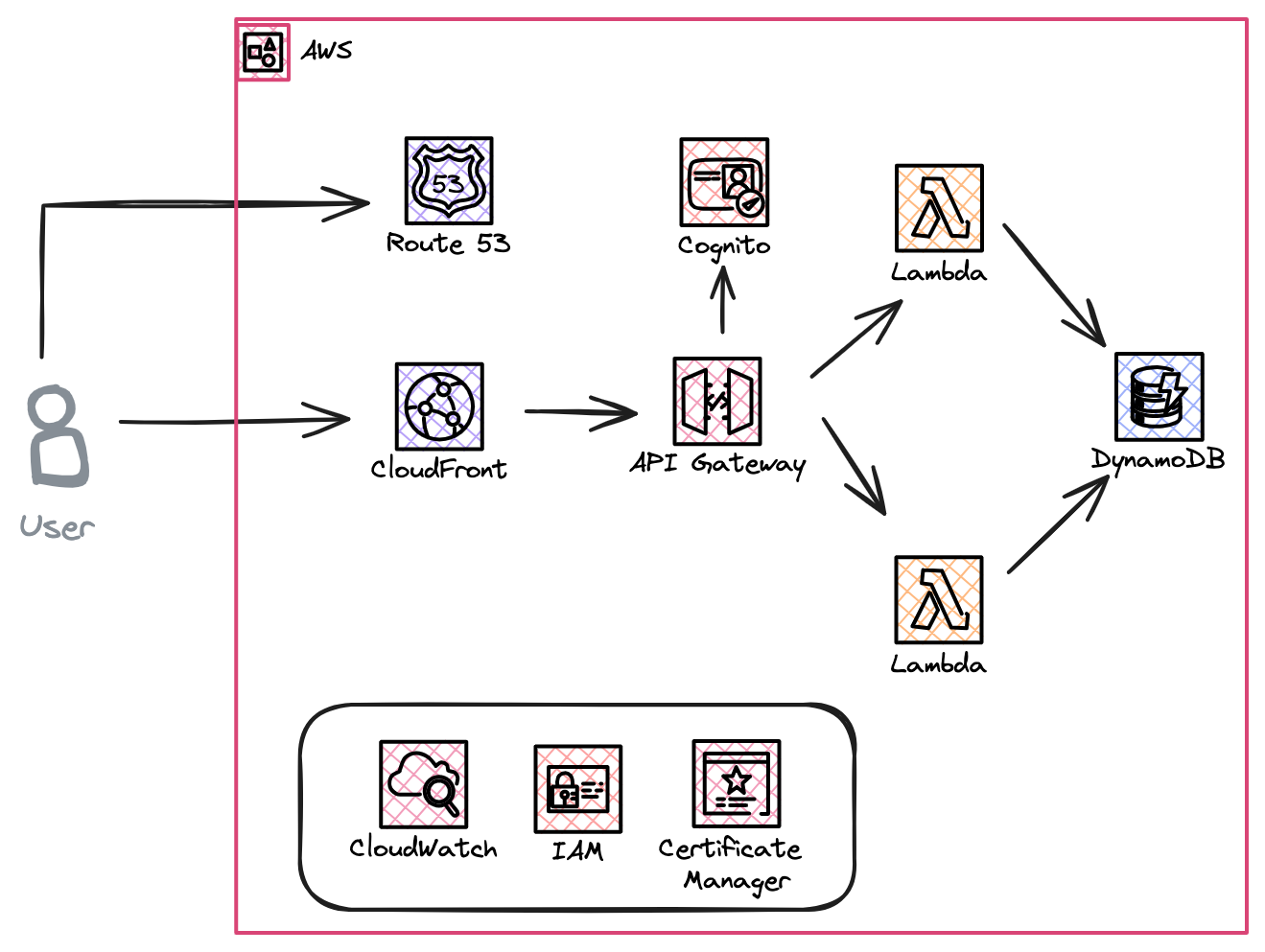
This architecture offers several benefits:
- No server management required
- Automatic scaling based on traffic
- Pay-per-use pricing model
- Quick deployment
Here's how the components interact:
- Users send requests to API Gateway
- API Gateway routes requests to the appropriate Lambda function
- For URL creation, Lambda generates a unique short code and stores the mapping in DynamoDB
- For URL redirection, Lambda first checks ElastiCache; if not found, it queries DynamoDB
- CloudWatch monitors the entire process and captures metrics
Day N Architecture: Scaling to Millions
As traffic grows, we can evolve our architecture to handle millions of users efficiently:
- Multi-Service Architecture:
- Frontend Service: Handles user interactions and initial request routing
- URL Creator Service: Specializes in generating short URLs
- URL Reader Service: Optimized for high-volume redirection requests
- Key Generation Service (KGS): Manages the creation of unique identifiers
- Data Management Service: Centralizes database operations
- Enhanced Infrastructure:
- Amazon CloudFront: Accelerates content delivery and caches popular redirects
- Amazon Elasticache in cluster mode: Distributes cache load across multiple nodes
- DynamoDB Global Tables: Replicates data across regions for global availability
- Amazon VPC with proper subnet isolation: Enhances security with public and private subnets
- Operational Improvements:
- Autoscaling Groups: Automatically adjusts capacity based on traffic patterns
- Multi-AZ deployment: Increases system resilience against zone failures
- AWS WAF and Shield: Protects against malicious traffic and DDoS attacks
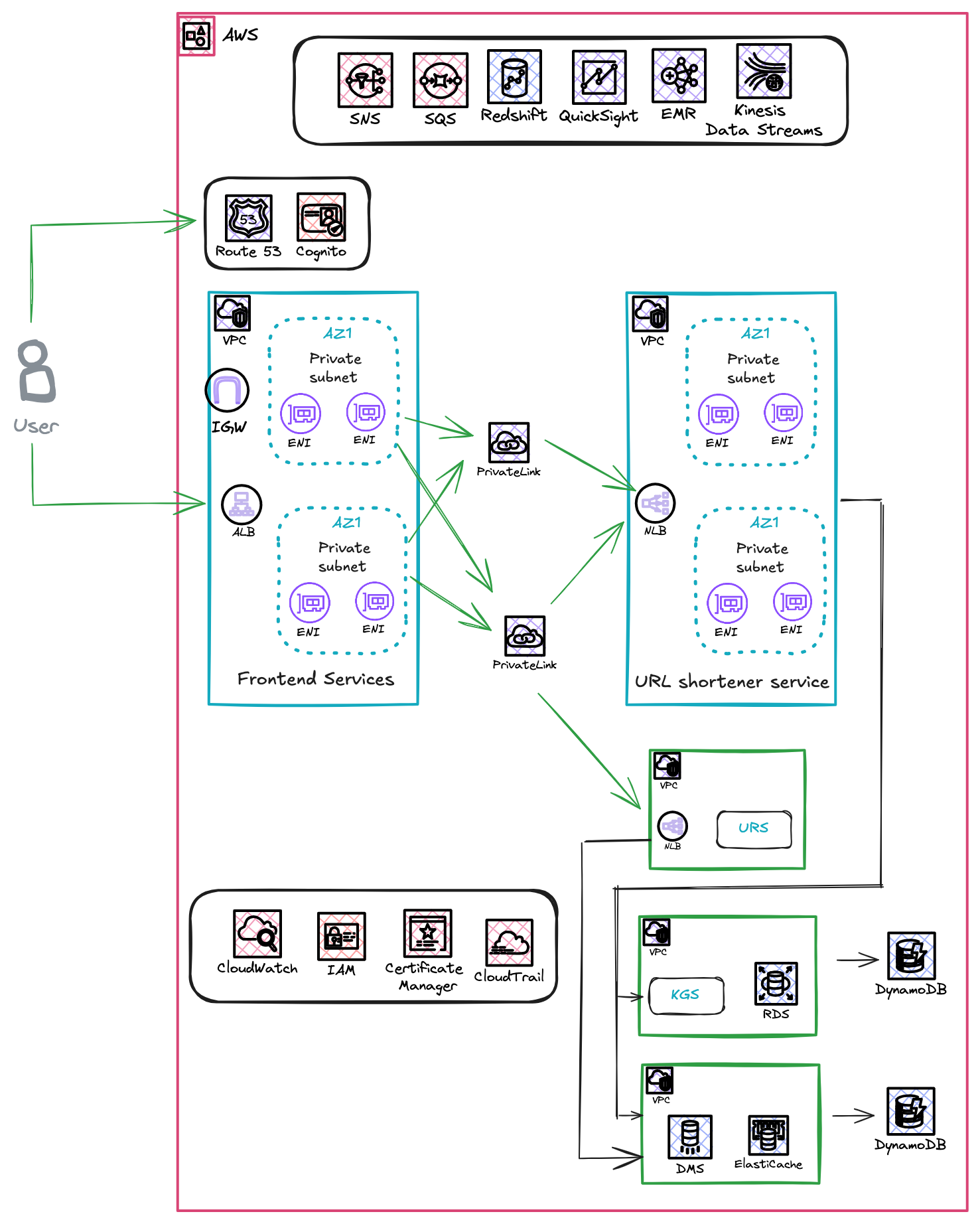
Database Considerations
The choice of database significantly impacts the system's performance and scalability. For our URL shortener, we'll use Amazon DynamoDB due to its:
- Horizontal scalability to handle billions of records
- Consistent single-digit millisecond performance
- Automatic data replication across multiple availability zones
- Support for time-to-live (TTL) for URL expiration
- Seamless integration with other AWS services
Our DynamoDB schema design:
Partition key = {short URL}
Sort key = SU (constant value)
For analytics and user-specific queries, we can implement a Global Secondary Index (GSI):
Partition key = {userId}
Sort key = u#{timestamp}
This design enables fast lookup of short URLs while also supporting analytics queries.
Implementation Details
Let's delve into specific implementation aspects of our URL shortener service.
API Design
Our service needs to support these core APIs:
- Create Short URL API:
POST /v1/createShortUrl
{
"longUrl": "https://example.com/very/long/url/path",
"customUrl": "myBrand", // Optional
"expiry": "2025-12-31", // Optional
"userMetadata": {} // Optional
}
- Redirect API:
GET v1/getLongUrl
{
"shortUrl": "abC7dEf",
"userMetadata": {} // Optional
}
Key Generation Service Implementation
Our KGS can be implemented as a separate microservice that:
- Pre-generates batches of unique IDs
- Stores them in a Redis cache for quick access
- Replenishes the pool when it falls below a threshold
Caching Strategy
To optimize performance, especially for popular URLs, we'll implement a multi-level caching strategy:
- API Gateway Cache: Caches responses at the edge for the most frequently accessed URLs
- Amazon CloudFront: Provides global edge caching for improved latency
- ElastiCache (Redis): Stores URL mappings with appropriate TTL settings
Our caching policy follows these principles:
- Cache popular URLs based on access frequency
- Implement cache invalidation when URLs expire or are deleted
- Use cache-aside pattern with DynamoDB as the source of truth
Advanced Features
Beyond the core functionality, we can enhance our URL shortener with advanced features:
Analytics Pipeline
To gain insights into URL usage patterns, we can implement an analytics pipeline:
- Capture click events and metadata in Amazon Kinesis Data Streams
- Process streams with Lambda or Kinesis Analytics
- Store aggregated metrics in Amazon S3 for long-term retention
- Visualize trends with Amazon QuickSight dashboards
This provides valuable data on:
- Most popular short URLs
- Geographic distribution of clicks
- Device and browser statistics
- Referral sources
Custom Domain Support
For enterprise customers who want branded short URLs, we can add multi-tenant support:
- Configure Route 53 to handle multiple custom domains
- Extend our DynamoDB schema to include tenant information
- Implement tenant-specific rate limiting and quotas
- Add custom branding options for redirect pages
Security Enhancements
To ensure the security of our service, we can implement:
- URL scanning for malicious content
- Rate limiting to prevent abuse
- AWS WAF rules to block suspicious patterns
- IP-based access controls for sensitive operations
Cost Optimization
One of the advantages of a serverless architecture is the ability to optimize costs based on actual usage. For our URL shortener service, we can implement these cost-saving measures:
- DynamoDB On-Demand Capacity: Start with on-demand pricing to match actual usage patterns
- Lambda Power Tuning: Optimize memory allocation for the best performance-to-cost ratio
- TTL for URL Expiration: Automatically remove expired URLs to reduce storage costs
- Reserved Capacity: For predictable workloads, purchase reserved capacity for significant savings
Performance Monitoring and Optimization
To ensure our service meets performance expectations, we'll implement comprehensive monitoring:
- CloudWatch Metrics: Track key performance indicators like latency, error rates, and request counts
- X-Ray Tracing: Identify bottlenecks in request processing
- CloudWatch Alarms: Set up alerts for operational issues
- CloudWatch Dashboard: Create a unified view of system performance
These monitoring tools help us identify opportunities for optimization, such as:
- Adjusting cache TTL settings based on access patterns
- Fine-tuning DynamoDB read/write capacity
- Optimizing Lambda function configurations
- Improving database query patterns
Deployment Strategy
For reliable deployments, we'll use infrastructure as code (IaC) with AWS CloudFormation or AWS CDK to define our entire architecture. This approach enables:
- Consistent environment creation across development, testing, and production
- Version-controlled infrastructure changes
- Automated testing of infrastructure modifications
- Blue-green deployment for zero-downtime updates
Conclusion
Building a scalable URL shortener service on AWS requires careful consideration of functional requirements, performance expectations, and scaling capabilities. By leveraging AWS's serverless and managed services, we can create a robust solution that scales from handling a few requests to billions of redirects.
The architecture we've outlined provides a solid foundation that can be implemented incrementally, starting with a simple Day 0 deployment and evolving toward a sophisticated multi-service architecture as demand grows. The serverless approach minimizes operational overhead while maximizing flexibility and cost-efficiency.
Key takeaways from our design include:
- Separating URL creation from redirection to optimize for different traffic patterns
- Using a dedicated Key Generation Service for reliable unique ID creation
- Implementing multi-level caching for performance optimization
- Building a scalable database design with DynamoDB
- Adding analytics capabilities for business insights
By following this guide, you can build a professional-grade URL shortening service that meets the needs of your organization or customers while leveraging the power and flexibility of AWS cloud services.