How DMA Frees Up Your CPU And Why It Matters
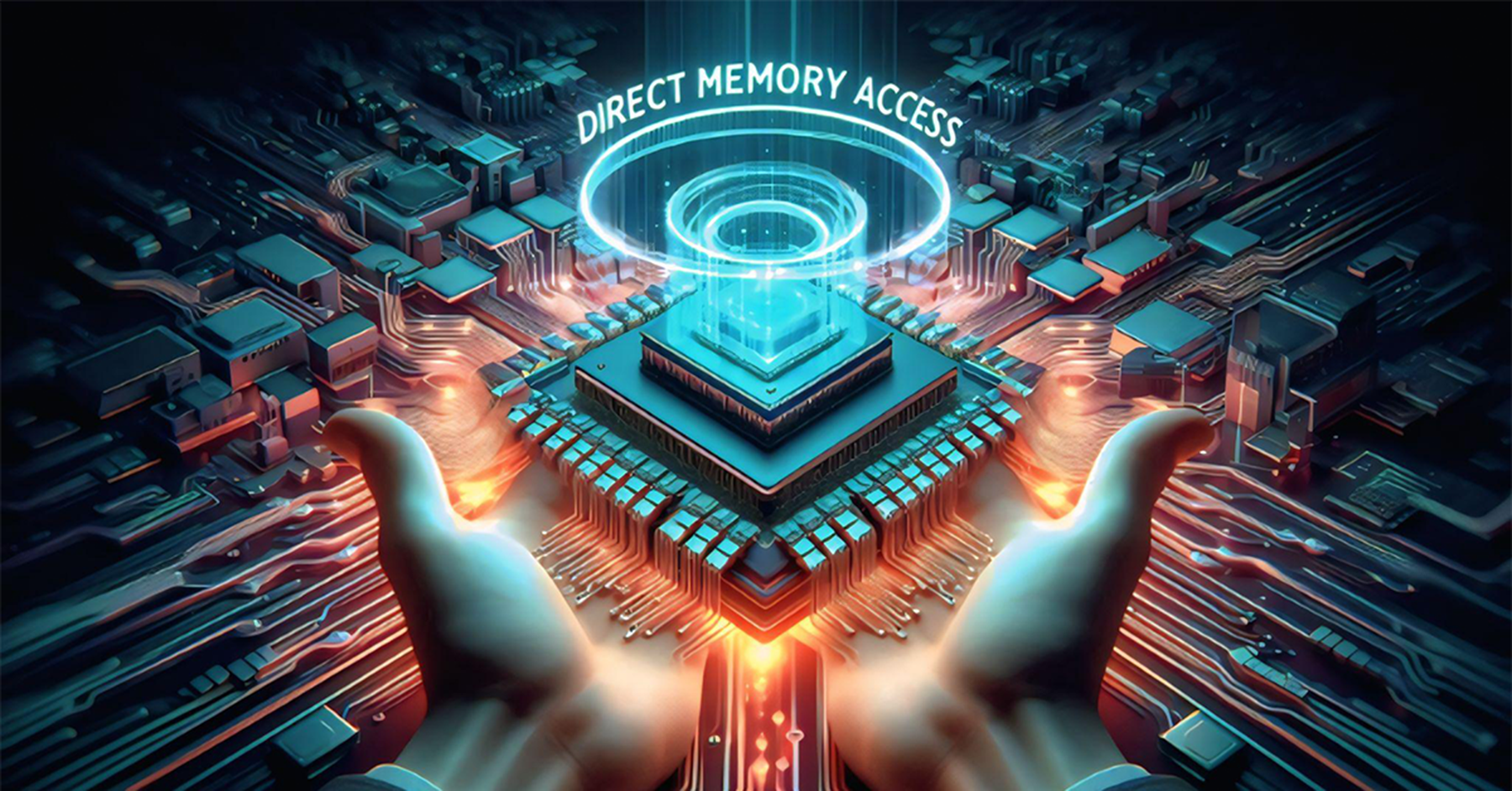
In embedded systems, efficiency hinges on managing large data volumes from sensors, communication modules, and storage interfaces while maintaining real-time performance. Direct Memory Access (DMA) tackles the bottleneck caused by CPU-managed data transfers by allowing peripherals to communicate directly with system memory, bypassing the CPU.
This offloading reduces CPU load, minimizes latency, and boosts throughput, critical for automotive, industrial, and IoT applications. Systems using DMA can achieve up to 10x faster data transfer speeds and reduce CPU load by as much as 70%, enhancing performance in high-speed sensor data acquisition, video streaming, and network processing.
What is DMA?
Direct Memory Access (DMA) is a hardware feature that allows peripherals to transfer data directly to and from system memory (RAM) without relying on the CPU. By bypassing the CPU, DMA reduces processing delays and ensures faster, more efficient data handling. This not only speeds up data transfer but also frees up the CPU to handle more complex tasks, improving overall system performance.
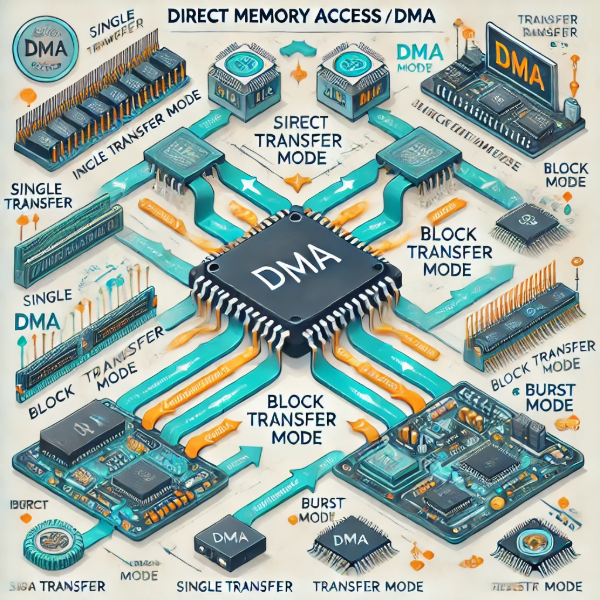
Key Benefits of DMA
Increased CPU Efficiency
DMA offloads data transfer tasks from the CPU, allowing it to focus on complex computations and real-time processing. In high-performance microcontrollers, DMA can reduce CPU load by up to 50% during continuous data acquisition.
High-Speed Transfers
DMA can transfer data at speeds limited only by memory and peripheral bandwidth. This is particularly useful for high-frequency ADC sampling or high-speed SPI/I2C communication. For example, STM32 microcontrollers using DMA for ADC sampling can achieve speeds of over 1 million samples per second, whereas DMA-based SPI transfers on the ESP32 can reach up to 80 Mbps — nearly 10x faster than CPU-based transfers.
Reduced Latency
Since DMA eliminates the need for CPU-mediated data handling, real-time response is faster and more reliable, a critical factor for automotive and industrial applications. For example, Automotive LiDAR systems rely on DMA to process sensor data with latencies below 1 ms. Also, ESP32-based DMA communication can reduce I/O latency by up to 40% compared to CPU-based handling.
DMA Transfer Modes
- Single Transfer Mode
- Transfers one word of data per request
- Suitable for low-frequency, small-scale data transfers
Example: Low-frequency sensor polling
- Block Transfer Mode
- Transfers a block of data continuously until the entire block is moved
- Useful for audio and video streaming
Example: DMA-based audio streaming can reach rates of up to 48 kHz for stereo audio
- Burst Mode
- Transfers multiple words of data in quick succession, minimizing bus access time and improving overall throughput
- Ideal for high-speed sensor data acquisition
Example: Burst mode in high-speed ADCs can achieve sampling rates exceeding 2 million samples per second
How DMA Works
DMA operation typically follows three stages:
1. Initialization
- The CPU configures the DMA controller with:
- Source and destination addresses
- Transfer size
- Transfer mode (single, block, or burst)
2. Data Transfer
- A peripheral (like an ADC) or memory block sends a transfer request to the DMA controller.
- The DMA controller takes control of the memory bus and executes the transfer.
- The CPU remains free for other tasks.
3. Completion
- Once the transfer is complete, the DMA controller generates an interrupt to notify the CPU.
- The CPU can then process the transferred data if needed.
In practical terms, this allows systems to sustain data transfer rates exceeding 100 Mbps without significant CPU involvement.
DMA is typically used for high-speed ADC, SPI, or I2C data transfers not for digital communication protocol
Let's take the DHT11 temperature sensor which uses a digital signal protocol rather than an ADC interface. It communicates through a single GPIO pin, which makes it easy to integrate but does not require DMA.
Practical Example: ESP32 ADC and DMA
Here’s an example of using the ESP32's DMA (Direct Memory Access) feature to capture data from an ADC (Analog-to-Digital Converter). This example demonstrates how to set up DMA to read sensor data efficiently.
Scenario
We’ll use an ESP32 ADC to continuously read data from a pressure sensor and store it in a buffer using DMA. This avoids CPU involvement during data transfer, ensuring efficient operation.
Program:
#include "driver/adc.h"
#include "driver/dma.h"
#include "esp_system.h"
#include "esp_log.h"
// Constants
#define ADC_CHANNEL ADC1_CHANNEL_0 // ADC channel for the sensor
#define ADC_WIDTH ADC_WIDTH_BIT_12 // ADC resolution (12 bits)
#define DMA_BUFFER_SIZE 512 // Size of the DMA buffer
// DMA buffer to store ADC readings
uint16_t dmaBuffer[DMA_BUFFER_SIZE];
// Tag for logging
const char* TAG = "DMA_ADC";
void setupADCandDMA() {
// Configure ADC
adc1_config_width(ADC_WIDTH);
adc1_config_channel_atten(ADC_CHANNEL, ADC_ATTEN_DB_11); // Set attenuation (0-3.6V range)
// Configure DMA for ADC
dma_config_t dmaConfig;
dmaConfig.direction = DMA_PERIPH_TO_MEMORY; // ADC to memory
dmaConfig.src_addr = (uint32_t)&(ADC1.apb_adc_out); // Source: ADC register
dmaConfig.dest_addr = (uint32_t)dmaBuffer; // Destination: buffer
dmaConfig.size = DMA_BUFFER_SIZE * sizeof(uint16_t); // Buffer size in bytes
dmaConfig.channel = 0; // DMA channel
dmaConfig.periph_inc = DMA_PINC_DISABLE; // Peripheral address doesn't increment
dmaConfig.mem_inc = DMA_MINC_ENABLE; // Memory address increments
dmaConfig.priority = DMA_PRIORITY_HIGH; // High priority
// Initialize DMA
if (dma_init(&dmaConfig) != ESP_OK) {
ESP_LOGE(TAG, "DMA initialization failed");
}
// Start DMA transfer
if (dma_start(&dmaConfig) != ESP_OK) {
ESP_LOGE(TAG, "DMA start failed");
}
}
void app_main() {
// Initialize ADC and DMA
steupADCandDMA();
// Main loop
while (true) {
// Simulate a delay to let DMA collect samples
vTaskDelay(pdMS_TO_TICKS(100));
// Process DMA data
for (int i = 0; i < DMA_BUFFER_SIZE; i++) {
float voltage = (dmaBuffer[i] / 4096.0) * 3.3; // Convert ADC value to voltage
ESP_LOGI(TAG, "ADC Value: %d, Voltage: %.2f V", dmaBuffer[i], voltage);
}
ESP_LOGI(TAG, "Processed DMA buffer data.");
}
}
Where DMA is Used
Embedded Systems
DMA can reduce CPU load by up to 40–50% in sensor-based systems
- High-speed data transfer from ADC, SPI, and I2C
- Continuous sensor data acquisition
Audio and Video Processing
DMA-based audio processing supports up to 192 kHz sample rates
- Streaming audio and video directly to memory
- Supports real-time encoding and decoding
Networking
NICs using DMA can sustain data rates of over 10 Gbps
- High-speed data handling in network interface cards (NICs)
Storage and Disk I/O
SSDs with DMA support can reach read/write speeds of over 3 GB/s
- Transfers data between storage controllers and memory
Automotive and IoT
LiDAR systems using DMA can generate over 1 million data points per second
- Automotive LiDAR, RADAR, and CAN bus for real-time decision-making
- IoT sensors for continuous environmental monitoring
DMA vs. CPU-Based Transfers
Feature | CPU-Based Transfers | DMA-Based Transfers |
Speed | Slower | Up to 10x faster |
CPU Load | High | Low (up to 70% reduction) |
Latency | Higher | Lower (up to 40% lower) |
Best for | Low-frequency, small data | High-speed, continuous data |
The Bottom Line
Direct Memory Access (DMA) transforms data handling in embedded systems by enabling fast, low-latency transfers without burdening the CPU. Whether it's streaming video, reading high-frequency sensor data, or processing network packets, DMA improves overall system efficiency and performance, making it a cornerstone of modern embedded design.
If you're aiming to optimize your embedded systems, connect to our experts now!