Google Introduces Agent Development Kit (ADK) at Cloud NEXT 2025
Learn How Developers Can Build Smart AI Agents with Google ADK
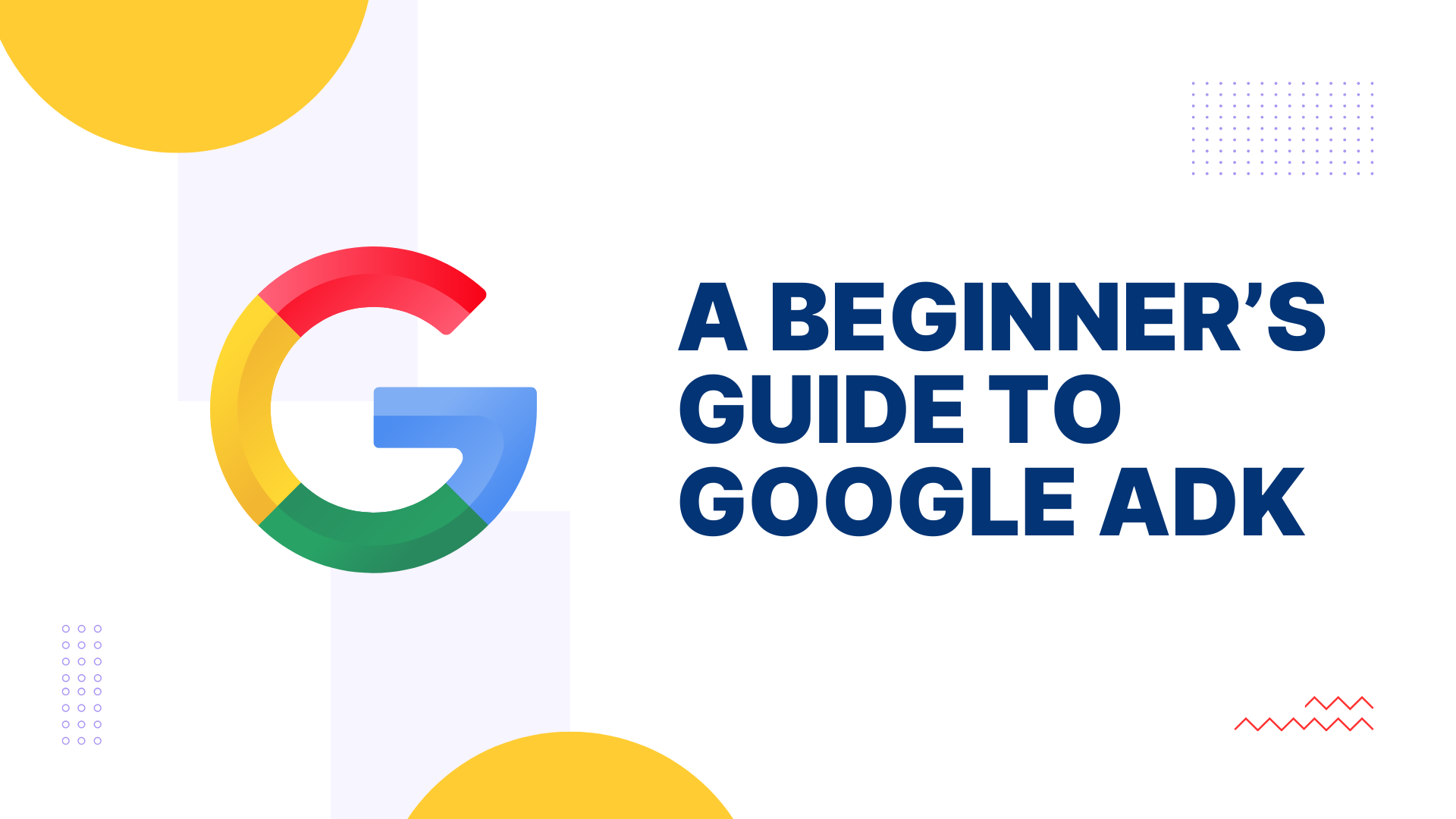
In a significant move aimed at shaping the future of AI development, Google announced the release of the Agent Development Kit (ADK) at its flagship event, Google Cloud NEXT 2025. Open-sourced and designed with developers in mind, ADK is a powerful new framework for building, evaluating, and deploying intelligent, production-grade AI agents and multi-agent systems.
Built on the same foundation powering Google’s own agentic applications, like Agentspace and the Customer Engagement Suite (CES), ADK brings the full lifecycle of agent development under one umbrella. Its introduction marks a clear step toward more autonomous, collaborative AI ecosystems beyond single-purpose models.
Moving from Single Agents to Collaborative Intelligence
As the GenAI space rapidly matures, developers are shifting focus from standalone bots to modular, multi-agent systems that can perform complex, coordinated tasks. ADK facilitates this transition with architectural flexibility, dynamic orchestration, and multi-model compatibility, all within a Pythonic, code-first framework.
“We’re at an inflection point where composability and collaboration between agents are the next frontier in AI. ADK is built to empower developers to build sophisticated agents with precise behavior and production readiness,” said a Google spokesperson during the announcement.
ADK’s Core Pillars
ADK’s architecture reflects a full-stack philosophy. It supports:
- Multi-Agent by Design: Developers can define hierarchies where agents delegate tasks intelligently based on context and capability.
- Model Flexibility: Use Google’s Gemini models, models from Vertex AI Model Garden, or third-party models via LiteLLM (Anthropic, Meta, AI21 Labs, Mistral, and more).
- Tool Integration: Agents can use pre-built tools like google_search and code_exec, or plug into ecosystems like LangChain and CrewAI.
- Streaming Support: Built-in bidirectional audio/video streaming enables more natural, multimodal interactions.
- Flexible Orchestration: Combine deterministic workflow agents with LLM-driven routing for adaptive and predictable behaviors.
- Developer-Centric Experience: Debug with CLI and a visual Web UI, inspect agent behavior step-by-step, and integrate easily with existing workflows.
A Real-World Use Case
One example presented at the event demonstrated ADK’s delegation capabilities using a WeatherAgent. The system comprised three agents: a root weather assistant, a greeting specialist, and a farewell handler.
With clear role descriptions and modular logic, the WeatherAgent can dynamically route tasks:
- A greeting like “Hi” is routed to the GreetingAgent.
- A weather query like “What’s the weather in Chicago?” is handled by the root agent using the get_weather tool.
- A farewell like “Bye” triggers the FarewellAgent.
This delegation level showcases ADK’s core principle: composability, where agents collaborate seamlessly through defined responsibilities.
How Does ADK Compare to Genkit?
For those exploring Google’s broader GenAI tooling, it’s important to differentiate ADK from Genkit:
Feature |
ADK |
Genkit
|
Focus |
Multi-agent
systems |
General-purpose
GenAI apps
|
Streaming |
Yes
(text, audio, video) |
Limited
|
Model
Integration |
Gemini,
Vertex AI, LiteLLM |
Gemini,
Vertex AI, plugins
|
Orchestration |
Built-in
workflows + LLM routing |
Plugin-based
|
Use
Case Fit |
Agent
hierarchies, dynamic routing |
Chatbots,
assistants, RAG
|
If you're building collaborative systems that require modular agent design, dynamic delegation, and fine-tuned control, ADK is purpose-built for your needs.
Prerequisites
Before we dive into building your first agent with the Google Agent Development Kit (ADK) and uv, let’s ensure you have everything you need to follow along. This section outlines the tools, accounts, and basic knowledge required to complete the project.
Required Tools and Accounts
- Python 3.9+
ADK requires Python 3.9 or higher. We’ll use uv to manage the Python version, so you don’t need Python pre-installed—uv can handle that for you. However, having a base Python installation (e.g., via your system package manager or python.org) simplifies the initial setup. - uv (Project Manager)
The star of our project management show! You’ll install uv as a standalone binary—no Python required upfront. We’ll cover the installation steps in the next section. - Google AI Studio API Key
To power our agent with the Gemini 2.0 Flash model, you’ll need an API key from Google AI Studio. Sign up or log in, navigate to the API section, and generate a key. We’ll configure it securely using environment variables. - Tavily API Key
For real-time web search, we’ll integrate the Tavily tool. It allows your agent to fetch fresh information from the web. You can get a free API key by signing up. - Text Editor or IDE
Use any editor you’re comfortable with—VS Code, PyCharm, or even Notepad++ will do the job. You’ll be writing Python and editing some configuration files.
Basic Knowledge Assumptions
This guide assumes you have:
- Basic Python Knowledge
You should be familiar with Python syntax, functions, and running scripts. You don’t need to be a pro—just comfortable enough to follow along. - Understanding of Virtual Environments
Knowing why and how virtual environments isolate dependencies is useful. If you’ve used venv, pipenv, or poetry, you’re good to go. - API Basics
You should know what an API key is and how it’s used to authenticate requests. No deep API experience required—we’ll guide you through the setup.
With these tools and basics in place, you’re ready to set up your project. In the next section, we’ll install uv, initialize your workspace, and add the necessary dependencies to get ADK up and running.
Getting Started
Installation is as simple as:
bash
CopyEdit
pip install google-adk
To create your first agent:
python
CopyEdit
from google.adk.agents import Agent
from google.adk.tools import google_search
root_agent = Agent(
name="search_assistant",
model="gemini-2.0-flash-exp",
instruction="You are a helpful assistant. Answer user questions using Google Search when needed.",
description="An assistant that can search the web.",
tools=[google_search]
)
Run it locally with:
bash
CopyEdit
adk run my_agent
Or explore the Web UI with:
bash
CopyEdit
adk web
Full
Full documentation, sample agents, and tutorials are available in the official ADK docs.
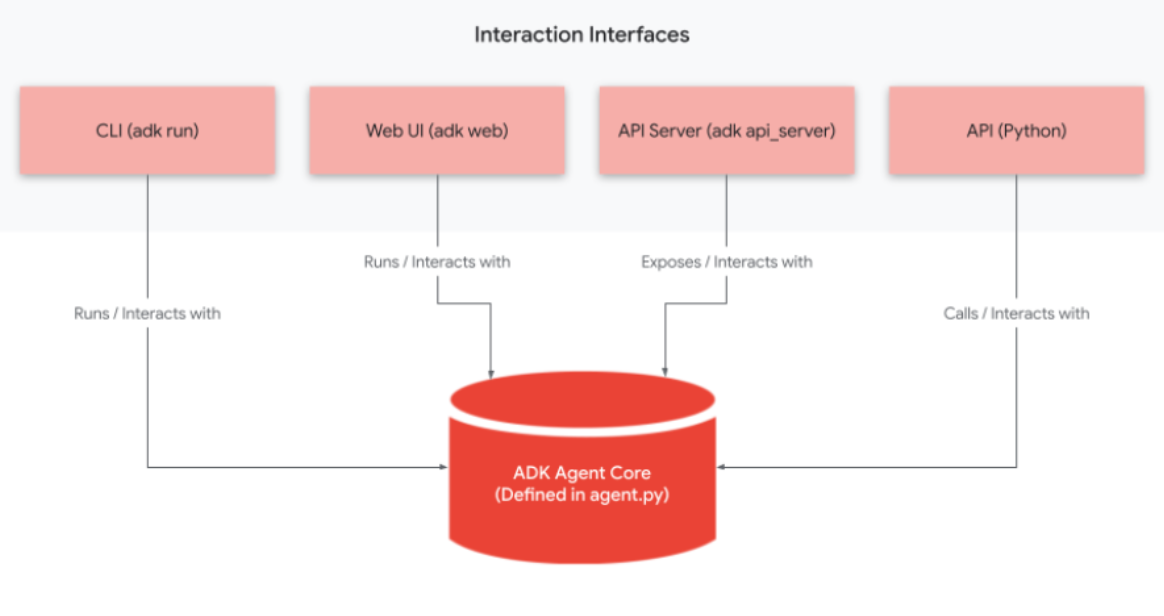
Building Multi-Agent Applications with ADK
While building a single-agent application is a great start, ADK truly shines when you orchestrate multiple agents to collaborate like a well-synced team. With ADK’s support for hierarchical agent structures and intelligent delegation, you can design systems where each agent specializes in a specific task, and hands off responsibility when appropriate.
Let’s walk through a practical example: a WeatherAgent that handles weather-related queries, but delegates greetings and farewells to dedicated sub-agents.
Step 1: Define a Tool
Agents rely on tools to perform tasks. Here, our WeatherAgent uses a tool to fetch weather information. ADK uses the function’s docstring to understand its purpose.
python
CopyEdit
def get_weather(city: str) -> Dict:
print(f"--- Tool: get_weather called for city: {city} ---")
city_normalized = city.lower().replace(" ", "")
mock_weather_db = {
"newyork": {"status": "success", "report": "The weather in New York is sunny with a temperature of 25°C."},
"london": {"status": "success", "report": "It's cloudy in London with a temperature of 15°C."},
"tokyo": {"status": "success", "report": "Tokyo is experiencing light rain and a temperature of 18°C."},
"chicago": {"status": "success", "report": "The weather in Chicago is sunny with a temperature of 25°C."},
"toronto": {"status": "success", "report": "It's partly cloudy in Toronto with a temperature of 30°C."},
"chennai": {"status": "success", "report": "It's rainy in Chennai with a temperature of 15°C."},
}
if city_normalized in mock_weather_db:
return mock_weather_db[city_normalized]
else:
return {"status": "error", "error_message": f"Sorry, I don't have weather information for '{city}'."}
Step 2: Define the Agents and Their Roles
Using LlmAgent, we define our specialized agents with clear instructions and capabilities.
python
CopyEdit
greeting_agent = Agent(
model=LiteLlm(model="anthropic/claude-3-sonnet-20240229"),
name="greeting_agent",
instruction="You are the Greeting Agent. Your ONLY task is to provide a friendly greeting to the user.",
description="Handles simple greetings and hellos",
)
farewell_agent = Agent(
model=LiteLlm(model="anthropic/claude-3-sonnet-20240229"),
name="farewell_agent",
instruction="You are the Farewell Agent. Your ONLY task is to provide a polite goodbye message.",
description="Handles simple farewells and goodbyes",
)
root_agent = Agent(
name="weather_agent_v2",
model="gemini-2.0-flash-exp",
description=(
"You are the main Weather Agent, coordinating a team.\n"
"- Your main task: Provide weather using the `get_weather` tool. "
"Handle its 'status' response ('report' or 'error_message').\n"
"- Delegation Rules:\n"
" • If the user gives a simple greeting (like 'Hi', 'Hello'), delegate to `greeting_agent`.\n"
" • If the user gives a simple farewell (like 'Bye', 'See you'), delegate to `farewell_agent`.\n"
"- Handle weather requests yourself using `get_weather`. For other queries, respond clearly if unsupported."
),
tools=[get_weather],
sub_agents=[greeting_agent, farewell_agent]
)
How Delegation Works
ADK’s LLM-powered routing determines whether a task should be handled by the current agent or delegated to a sub-agent. This decision is based on:
- The current agent’s role and instructions
- The sub-agents’ descriptions
- The intent behind the user’s input
For example:
- A message like “Hi” triggers a delegation to GreetingAgent.
- A message like “What’s the weather in Chicago?” is handled directly by the root WeatherAgent.
- Clear, distinct descriptions are critical for accurate routing.
This approach allows you to build modular, maintainable agent systems that mimic how human teams divide and conquer tasks.
Evaluation and Deployment
Building a smart agent is only half the battle, reliability and scalability are what bring it to life in the real world. ADK includes:
Evaluation
Use AgentEvaluator.evaluate() to programmatically test your agent’s behavior against predefined datasets (e.g., evaluation.test.json). You can also:
- Run evaluations via the CLI using adk eval
- Use ADK’s web UI for visual inspection and test management
These tools help ensure your agents behave as expected across typical and edge-case scenarios.
Deployment
Once validated, your agent can be deployed in multiple ways:
- Container Runtime: Package and deploy your agent like any modern microservice.
- Vertex AI Agent Builder: ADK integrates with Google Cloud’s managed runtime for scalability, monitoring, and enterprise-grade support.
This production-ready deployment path allows you to confidently transition from prototype to production. ADK also supports over 100 pre-built connectors, enabling agents to interact with enterprise systems like BigQuery, AlloyDB, NetApp, or APIs managed via Apigee.
Security and Governance
For enterprise-grade deployments, the Agent Development Kit (ADK) includes a range of built-in safeguards designed to enhance control, visibility, and trust:
- Response Moderation
Built-in output controls help moderate agent responses and prevent inappropriate or unintended outputs. - Scoped Identity Permissions
Granular permission settings allow you to restrict what agents can access or perform, aligning with organizational security policies. - Input Screening
Automated input validation and filtering help catch potentially harmful or malformed inputs before they reach your agent. - Behavior Monitoring and Audit Logs
Comprehensive logging capabilities allow teams to track agent behavior, enabling audits and supporting compliance requirements.
Together, these capabilities help enterprises deploy AI agents more safely and confidently, especially in regulated or sensitive environments.
ADK Works Anywhere—But It's Optimized for Google Cloud
The Agent Development Kit (ADK) is designed to be platform-agnostic, offering flexibility to run agents across environments. However, its full potential is unlocked when used within the Google Cloud ecosystem.
Deep Integration with Gemini and Vertex AI
ADK is purpose-built to work seamlessly with Gemini models, especially advanced variants like Gemini 2.5 Pro Experimental, known for superior reasoning and tool execution. When paired with Vertex AI, ADK provides a native deployment path to an enterprise-grade, fully managed runtime with built-in scalability, monitoring, and reliability.
Enterprise Connectivity
Beyond model integration, ADK enables powerful connectivity across your enterprise stack:
- 100+ Pre-Built Connectors: Agents can directly interface with enterprise systems and third-party tools without manual wiring.
- Workflow Integration: Leverage workflows built with Application Integration to orchestrate business processes.
- Direct Data Access: Query data from BigQuery, AlloyDB, NetApp, and more—no duplication required.
API-First Design
Agents can also interact with internal APIs managed via Apigee, letting them securely reuse your organization’s existing API infrastructure and governance policies.
Conclusion
By open-sourcing ADK, Google is not just enabling developers, it’s signaling a strategic direction. AI is no longer about individual, powerful models; it’s about how they collaborate, adapt, and reason across contexts. ADK is Google’s blueprint for building the next generation of agentic experiences, at scale and in production.
Whether you're building a team of task-specific agents or orchestrating enterprise-scale automation, ADK is ready to meet you at the cutting edge.